Checkboxes field
The checkboxes field stores an array of strings representing multiple choices.
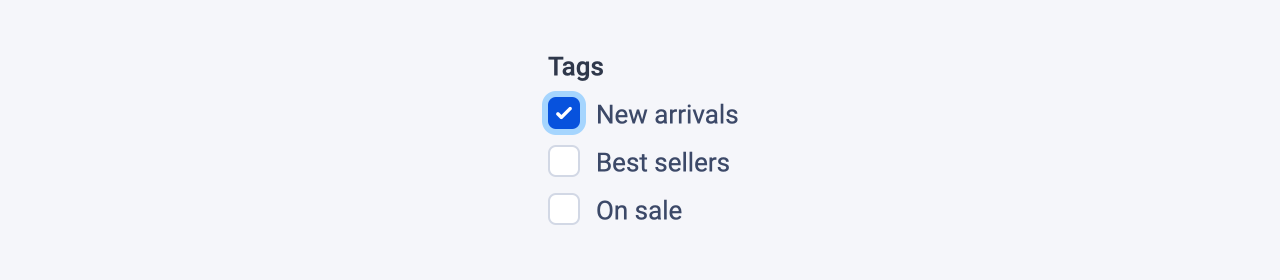
Examples
Here's an example of using the checkboxes field in a block:
# blocks/IconBox.vue
<template>
<div>
<div v-if="tags?.length" class="flex gap-2">
<span v-for="tag in tags">{{ choices[tag] }}</span>
</div>
...
</div>
</template>
<script lang="ts" setup>
import { checkboxesField } from '#pruvious'
const choices = {
'new-arrivals': 'New arrivals',
'best-sellers': 'Best sellers',
'on-sale': 'On sale',
}
defineProps({
tags: checkboxesField({
choices,
default: ['new-arrivals'],
}),
})
</script>
Here is another example of how to use it in a collection definition:
# collections/products.ts
import { defineCollection } from '#pruvious'
export default defineCollection({
name: 'products',
mode: 'multi',
fields: {
tags: {
type: 'checkboxes',
options: {
choices: {
'new-arrivals': 'New arrivals',
'best-sellers': 'Best sellers',
'on-sale': 'On sale',
},
default: ['new-arrivals'],
},
},
// ...
},
})
Options
You can specify the following options in the checkboxes field (required fields are marked with the symbol R):
Option | Description |
---|---|
| A key-value object containing permissible choices, where the key represents the choice value, and the value represents the corresponding checkbox label. |
| The default field value ( |
| A brief descriptive text displayed in code comments and in a tooltip at the upper right corner of the field. |
| The field label displayed in the UI. By default, it is automatically generated based on the property name assigned to the field. For example, availableSizes becomes Available sizes. |
| A string that specifies the |
| A stringified TypeScript type used for overriding the automatically generated field value type. This feature is only applicable when declaring the field in a collection. |
| Indicates whether the field input is mandatory, meaning it must be present during creation, and at least one value must be selected. |
| Indicates whether the checkboxes are sortable. |
Last updated on January 6, 2024 at 14:00