Text field
The text field is the most frequently used field for storing strings.
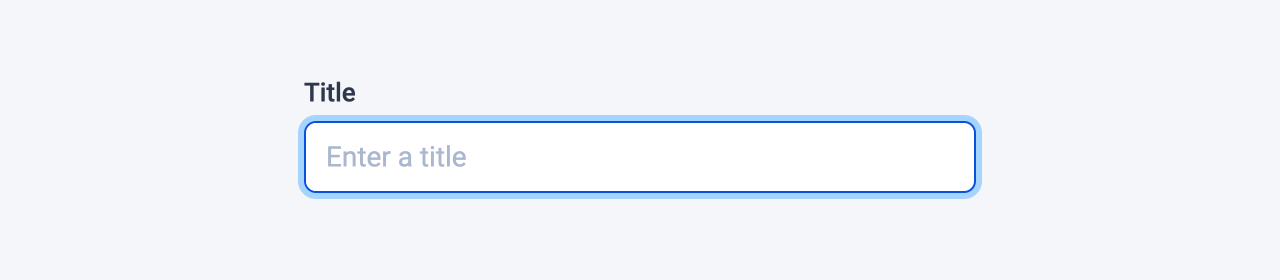
Examples
Here's an example of using the text field in a block:
# blocks/Section.vue
<template>
<div>
<h2 v-if="title">{{ title }}</h2>
<slot />
</div>
</template>
<script lang="ts" setup>
import { textField } from '#pruvious'
defineProps({
title: textField({
placeholder: 'Enter a title',
}),
})
</script>
Here is another example of how to use it in a collection definition:
# collections/subscribers.ts
import { defineCollection } from '#pruvious'
import { emailValidator, uniqueValidator } from '#pruvious/server'
export default defineCollection({
name: 'subscribers',
mode: 'multi',
dashboard: { icon: 'Mail' },
fields: {
email: {
type: 'text',
options: {
label: 'Email address',
required: true,
type: 'email',
autocomplete: 'email',
},
additional: {
index: true,
unique: 'allLanguages',
validators: [emailValidator, uniqueValidator],
},
},
},
})
Options
You can specify the following options in the text field:
Option | Description |
---|---|
| A string specifying autocomplete behavior for the input element. Defaults to |
| A boolean indicating whether to display a clear button that removes the current input text. |
| The default field value (an empty string if not defined). |
| A brief descriptive text displayed in code comments and in a tooltip at the upper right corner of the field. |
| The field label displayed in the UI. By default, it is automatically generated based on the property name assigned to the field. For example, firstName becomes First name. |
| A string that specifies the |
| A stringified TypeScript type used for overriding the automatically generated field value type. This feature is only applicable when declaring the field in a collection. |
| Text that appears in the input element when it has no value set. |
| A short text to be prepended before the input element. |
| Specifies that the input field is mandatory during creation and cannot have an empty string value. |
| A booleanish value indicating whether spellchecking is enabled for the input element. Defaults to |
| A short text to be appended after the input element. |
| Specifies whether to remove whitespace from both ends of the input string. |
| Input |
Last updated on January 5, 2024 at 20:10