Records field
The records field stores relationships to multiple records from a specified collection as an array of integers.
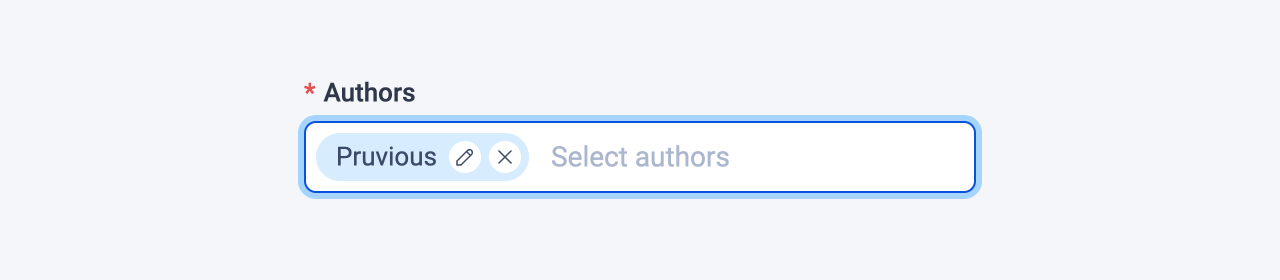
Examples
Here's an example of using the records field in a block:
# blocks/Article.vue
<template>
<div>
<span>Authors:</span>
<span v-for="author of authors">{{ author?.firstName }} {{ author?.lastName }}</span>
...
</div>
</template>
<script lang="ts" setup>
import { recordsField } from '#pruvious'
defineProps({
authors: recordsField({
collection: 'users',
fields: { firstName: true, lastName: true },
required: true,
placeholder: 'Select authors',
}),
})
</script>
Here is another example of how to use it in a collection definition:
# collections/posts.ts
import { defineCollection } from '#pruvious'
export default defineCollection({
name: 'posts',
mode: 'multi',
fields: {
authors: {
type: 'records',
options: {
collection: 'users',
fields: { firstName: true, lastName: true, email: true },
required: true,
placeholder: 'Select authors',
},
},
// ...
},
})
Options
You can specify the following options in the records field (required fields are marked with the symbol R):
Option | Description |
---|---|
| The name of the multi-entry collection from which to retrieve the records. |
| The default field value ( |
| A brief descriptive text displayed in code comments and in a tooltip at the upper right corner of the field. |
| An array of field names from the selected collection to display in tooltips. |
| The fields of the selected collection to be returned when this field's values are populated. Defaults to |
| The field label displayed in the UI. By default, it is automatically generated based on the property name assigned to the field. For example, categories becomes Categories. |
| A string that specifies the |
| Text that appears in the search input when there is no value. |
| Specifies whether to populate the fields of the selected collection. Exercise caution when using this option, as it may trigger infinite loops during population if the related collection fields depend on additional population loops. Defaults to |
| The collection field or fields used as the record label. When using multiple fields, the first field is used as the main label, and the second field is displayed only in search results. By default, the fields from the |
| Indicates whether the field input is mandatory, meaning it must be present during creation, and at least one record must be selected. |
| Indicates whether the records are sortable. Defaults to |
| The number of visible choices in the dropdown list (must be less than 30). |
Last updated on January 9, 2024 at 11:57