Repeater field
The repeater field stores an array of subfield values.
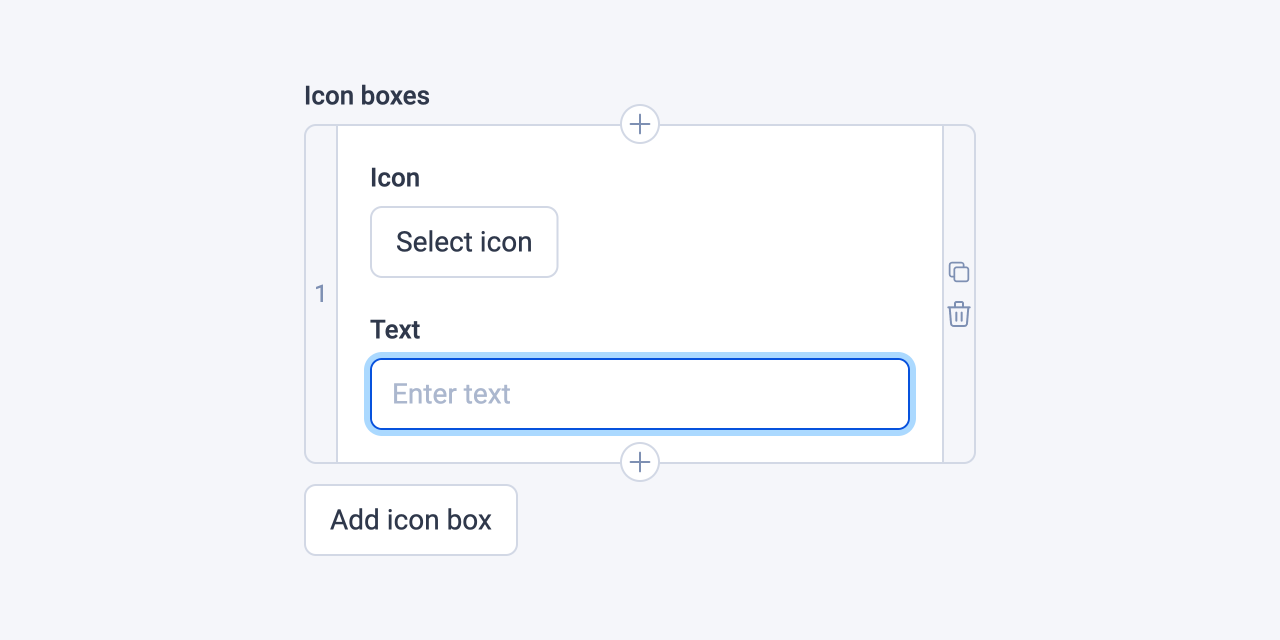
Examples
Here's an example of using the repeater field in a block:
# blocks/IconBoxes.vue
<template>
<div class="grid grid-cols-3">
<div v-for="{ icon, text } of iconBoxes">
<PruviousIcon :icon="icon" />
<p>{{ text }}</p>
</div>
</div>
</template>
<script lang="ts" setup>
import { iconSubfield, repeaterField, textAreaSubfield } from '#pruvious'
defineProps({
iconBoxes: repeaterField({
subfields: {
icon: iconSubfield(),
text: textAreaSubfield({ placeholder: 'Enter text' }),
},
addLabel: 'Add icon box',
}),
})
</script>
Here is another example of how to use it in a collection definition:
# collections/products.ts
import { defineCollection } from '#pruvious'
export default defineCollection({
name: 'products',
mode: 'multi',
fields: {
variations: {
type: 'repeater',
options: {
subfields: {
size: {
type: 'button-group',
options: {
choices: {
S: 'Small',
M: 'Medium',
L: 'Large',
},
},
},
available: {
type: 'switch',
options: {},
},
},
fieldLayout: ['size', 'available'],
addLabel: 'Add variation',
},
},
},
})
Options
You can specify the following options in the repeater field (required fields are marked with the symbol R):
Option | Description |
---|---|
| The text label displayed on the button used to add a new repeater item. Defaults to |
| The default field value ( |
| A brief descriptive text displayed in code comments and in a tooltip at the upper right corner of the field. |
| Defines the field layout in the repeater. The layout array accepts the following values:
If not specified, all displayable subfields will be shown vertically one after another. |
| The field label displayed in the UI. By default, it is automatically generated based on the property name assigned to the field. For example, productVariations becomes Product variations. |
| The maximum allowed number of repeater entries. |
| The minimum allowed number of repeater entries. Defaults to |
| A stringified TypeScript type used for overriding the automatically generated field value type. This feature is only applicable when declaring the field in a collection. |
| Specifies that the field input is mandatory during creation, requiring at least one entry. |
| An object of subfields that define the structure of each repeater entry. |
Last updated on January 9, 2024 at 11:50