Editor field
The editor field contains a rich text editor that stores text content in HTML format.
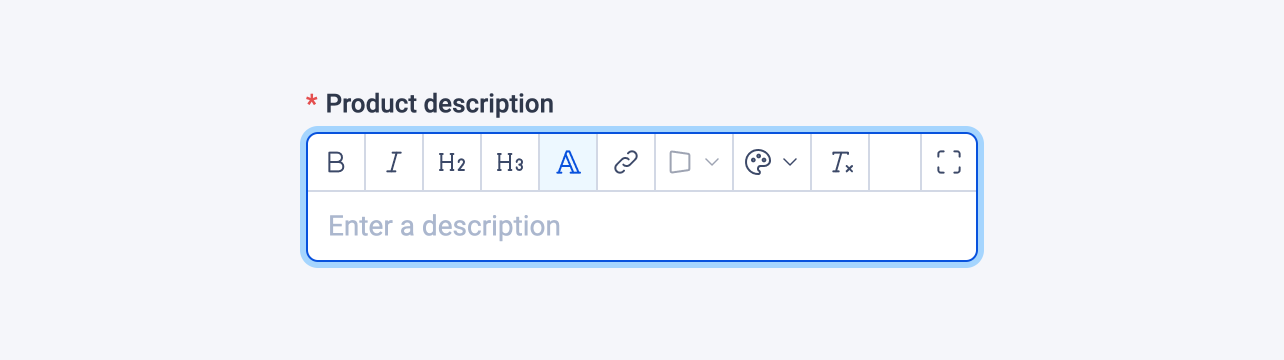
Examples
Here's an example of using the editor field in a block:
# blocks/Product.vue
<template>
<PruviousHTML :html="description" />
</template>
<script lang="ts" setup>
import { editorField } from '#pruvious'
defineProps({
description: editorField({
label: 'Product description',
placeholder: 'Enter a description',
blockFormats: [{ className: 'p-6 border rounded', label: 'Boxed', tags: ['div'] }],
inlineFormats: [{ className: 'text-red-500', label: 'Red' }],
toolbar: ['bold', 'italic', 'heading2', 'heading3', 'paragraph', 'link', 'blockFormats', 'inlineFormats', 'clear'],
required: true,
}),
})
</script>
Here is another example of how to use it in a collection definition:
# collections/products.ts
import { defineCollection } from '#pruvious'
export default defineCollection({
name: 'products',
mode: 'multi',
fields: {
description: {
type: 'editor',
options: {
label: 'Product description',
placeholder: 'Enter a description',
blockFormats: [{ className: 'p-6 border rounded', label: 'Boxed', tags: ['div'] }],
inlineFormats: [{ className: 'text-red-500', label: 'Red' }],
toolbar: [
'bold',
'italic',
'heading2',
'heading3',
'paragraph',
'link',
'blockFormats',
'inlineFormats',
'clear',
],
required: true,
},
},
// ...
},
})
Options
You can specify the following options in the editor field:
Option | Description |
---|---|
| Specifies whether to allow the editor to enter fullscreen mode. Defaults to |
| An array of objects that specifies the block formats to display in the toolbar. Block formats are used to specify CSS classes to be applied to the block element. They are displayed as a dropdown list in the toolbar. |
| The default field value ( |
| A brief descriptive text displayed in code comments and in a tooltip at the upper right corner of the field. |
| An array of objects that specifies the inline formats to display in the toolbar. Inline formats are used to specify CSS classes to be applied to the inline element. They are displayed as a dropdown list in the toolbar. |
| The field label displayed in the UI. By default, it is automatically generated based on the property name assigned to the field. For example, heroContent becomes Hero content. |
| Text that appears in the input element when it has no value set. |
| Specifies that the field input is mandatory during creation, and the field value cannot be empty. |
| An array of strings that specifies the toolbar buttons to display. The available buttons are as follows:
|
Last updated on January 7, 2024 at 16:17