Time range field
The time range field stores a tuple of timestamps in milliseconds that represent two time values.
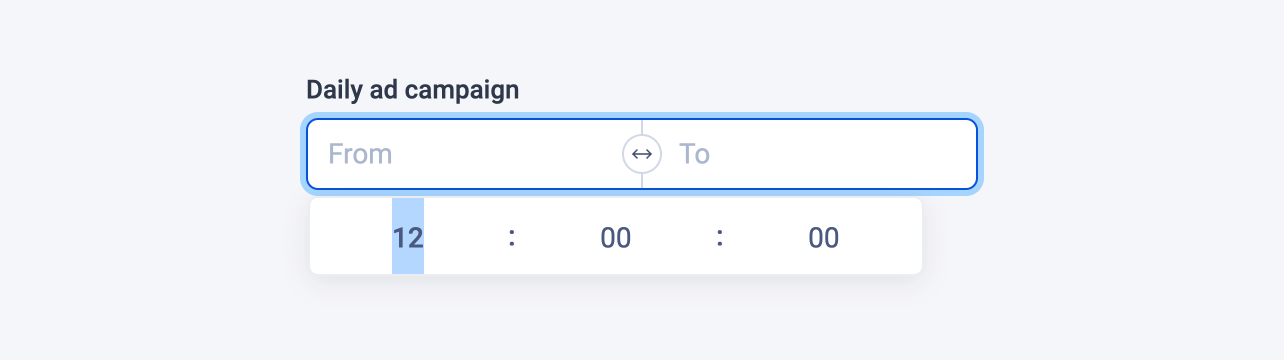
Examples
Here's an example of using the time range field in a block:
# blocks/ProductAd.vue
<template>
<div v-if="showAds">
...
</div>
</template>
<script lang="ts" setup>
import { timeRangeField } from '#pruvious'
const props = defineProps({
dailyAdCampaign: timeRangeField({
placeholder: ['From', 'To'],
}),
})
const showAds = computed(() => {
const now = Date.now()
return (
props.dailyAdCampaign?.[0] &&
props.dailyAdCampaign?.[1] &&
now >= props.dailyAdCampaign[0] &&
now <= props.dailyAdCampaign[1]
)
})
</script>
Here is another example of how to use it in a collection definition:
# collections/products.ts
import { defineCollection } from '#pruvious'
export default defineCollection({
name: 'products',
mode: 'multi',
fields: {
dailyAdCampaign: {
type: 'time-range',
options: {
placeholder: ['From', 'To'],
},
},
// ...
},
})
Options
You can specify the following options in the time range field:
Option | Description |
---|---|
| A boolean indicating whether to display a clear button that removes the current value. The clearable option can be defined as a tuple of booleans, with each boolean representing a picker. |
| The default field value ( |
| A brief descriptive text displayed in code comments and in a tooltip at the upper right corner of the field. |
| The field label displayed in the UI. By default, it is automatically generated based on the property name assigned to the field. For example, eventTime becomes Event time. |
| The latest possible time (as timestamp in milliseconds). This value should not exceed 86399999 ms. |
| The earliest possible time (as timestamp in milliseconds). |
| A string that specifies the |
| Text that appears in the input elements when they have no value set. You can specify the placeholder as a tuple of strings. |
| Specifies that the field input is mandatory during creation. |
Last updated on January 6, 2024 at 17:56