Size field
The size field stores an object that includes numeric values along with their corresponding units.
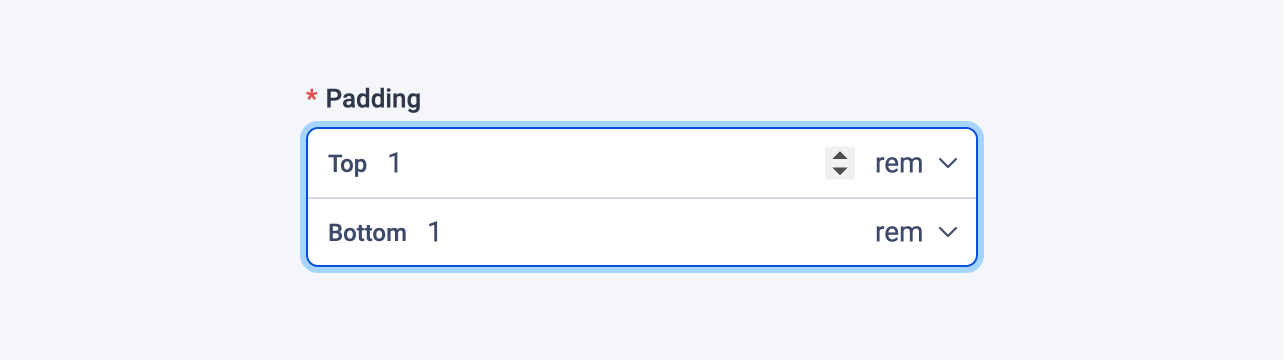
Examples
Here's an example of using the size field in a block:
# blocks/Container.vue
<template>
<div
:style="{
paddingTop: `${padding?.top.value}${padding?.top.unit}`,
paddingBottom: `${padding?.bottom.value}${padding?.bottom.unit}`,
}"
>
...
</div>
</template>
<script lang="ts" setup>
import { sizeField } from '#pruvious'
defineProps({
padding: sizeField({
required: true,
inputs: {
top: { min: 0, units: ['px', 'rem'] },
bottom: { min: 0, units: ['px', 'rem'] },
},
default: {
top: { value: 1, unit: 'rem' },
bottom: { value: 1, unit: 'rem' },
},
}),
})
</script>
Here is another example of how to use it in a collection definition:
# collections/products.ts
export default defineCollection({
name: 'products',
mode: 'multi',
fields: {
dimensions: {
type: 'size',
options: {
required: true,
inputs: {
width: {
units: ['cm', 'in'],
},
height: {
units: ['cm', 'in'],
},
},
},
},
// ...
},
})
Options
You can specify the following options in the size field:
Option | Description |
---|---|
| The default field value. By default, all defined size |
| A brief descriptive text displayed in code comments and in a tooltip at the upper right corner of the field. |
| A record of subfields that make up the field. The keys of the record are used as the subfield names. Defaults to |
| The field label displayed in the UI. By default, it is automatically generated based on the property name assigned to the field. For example, imageSize becomes Image size. |
| A string that specifies the |
| Specifies that the field input is mandatory during creation. |
| Whether the size values can be synchronized in the field UI. Defaults to |
Last updated on January 9, 2024 at 11:09