File field
The file field stores a relationship to a record in the uploads collection.
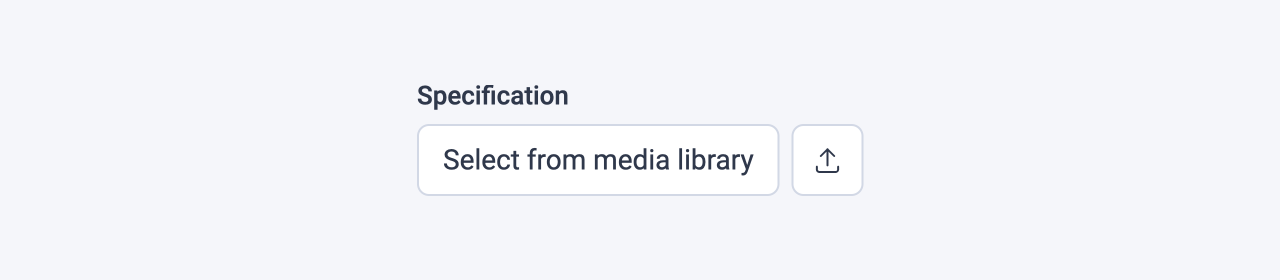
Examples
Here's an example of using the file field in a block:
# blocks/Product.vue
<template>
<div>
<a
v-if="specification"
:href="getPublicFilePath(specification, runtimeConfig)"
download
target="_blank"
>
Download specification
</a>
...
</div>
</template>
<script lang="ts" setup>
import { fileField, getPublicFilePath } from '#pruvious'
defineProps({
specification: fileField({
allowedTypes: ['pdf'],
}),
})
const runtimeConfig = useRuntimeConfig()
</script>
Here is another example of how to use it in a collection definition:
# collections/products.ts
import { defineCollection } from '#pruvious'
export default defineCollection({
name: 'products',
mode: 'multi',
fields: {
specification: {
type: 'file',
options: {
allowedTypes: ['pdf'],
},
},
// ...
},
})
Options
You can specify the following options in the file field:
Option | Description |
---|---|
| An array of allowed file types or extensions (e.g., |
| The default field value ( |
| A brief descriptive text displayed in code comments and in a tooltip at the upper right corner of the field. |
| The fields of the uploads collection to be returned when this field's value is populated. Defaults to |
| The field label displayed in the UI. By default, it is automatically generated based on the property name assigned to the field. For example, productVideo becomes Product video. |
| The maximum allowed file size in bytes. You can provide this limit as a string (e.g., |
| A string that specifies the |
| Specifies whether to populate the fields of the uploads collection. By default, the collection does not have fields that can be populated. Defaults to |
| Specifies that the field input is mandatory during creation. |
Last updated on January 7, 2024 at 17:16