Image field
The file field stores a relationship to an image record in the uploads collection.
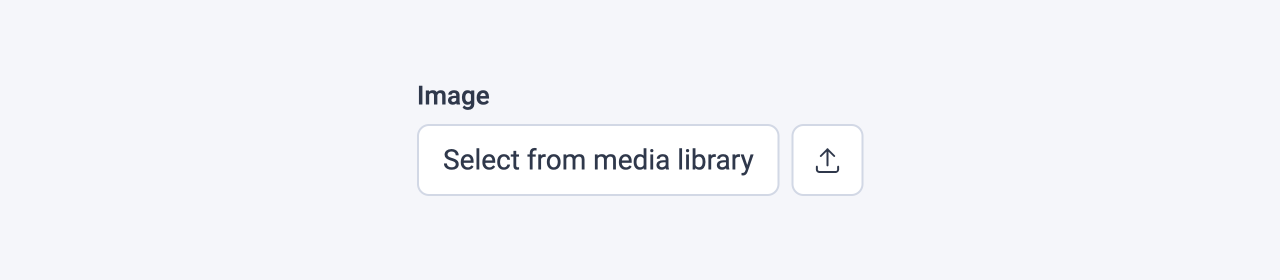
Examples
Here's an example of using the image field in a block:
# blocks/Product.vue
<template>
<div>
<PruviousPicture :image="image" :lazy="true" />
...
</div>
</template>
<script lang="ts" setup>
import { imageField } from '#pruvious'
defineProps({
image: imageField({
minWidth: 1600,
minHeight: 1600,
sources: [
{ media: '(max-width: 768px)', format: 'webp', width: 1024, height: 1024, resize: 'cover' },
{ media: '(max-width: 768px)', format: 'jpeg', width: 1024, height: 1024, resize: 'cover' },
{ format: 'webp', width: 1600, height: 1600, resize: 'cover' },
{ format: 'jpeg', width: 1600, height: 1600, resize: 'cover' },
],
}),
})
</script>
Here is another example of how to use it in a collection definition:
# collections/products.ts
import { defineCollection } from '#pruvious'
export default defineCollection({
name: 'products',
mode: 'multi',
fields: {
image: {
type: 'image',
options: {
minWidth: 1600,
minHeight: 1600,
sources: [
{ media: '(max-width: 768px)', format: 'webp', width: 1024, height: 1024, resize: 'cover' },
{ media: '(max-width: 768px)', format: 'jpeg', width: 1024, height: 1024, resize: 'cover' },
{ format: 'webp', width: 1600, height: 1600, resize: 'cover' },
{ format: 'jpeg', width: 1600, height: 1600, resize: 'cover' },
],
},
// ...
},
})
Options
You can specify the following options in the image field:
Option | Description |
---|---|
| An array of allowed image types or extensions (e.g., |
| The default field value ( |
| A brief descriptive text displayed in code comments and in a tooltip at the upper right corner of the field. |
| The field label displayed in the UI. By default, it is automatically generated based on the property name assigned to the field. For example, productImage becomes Product image. |
| The minimum allowed image height in pixels. |
| The minimum allowed image width in pixels. |
| A string that specifies the |
| Specifies that the field input is mandatory during creation. |
| An array of optimized image sources. When this field is populated, the resulting object will include a
|
| Specifies whether to transform SVG images. If |
Last updated on January 7, 2024 at 17:44